Understanding Decision Trees in Supervised Learning
- vazquezgz
- Oct 15, 2023
- 8 min read
Updated: Mar 4, 2024
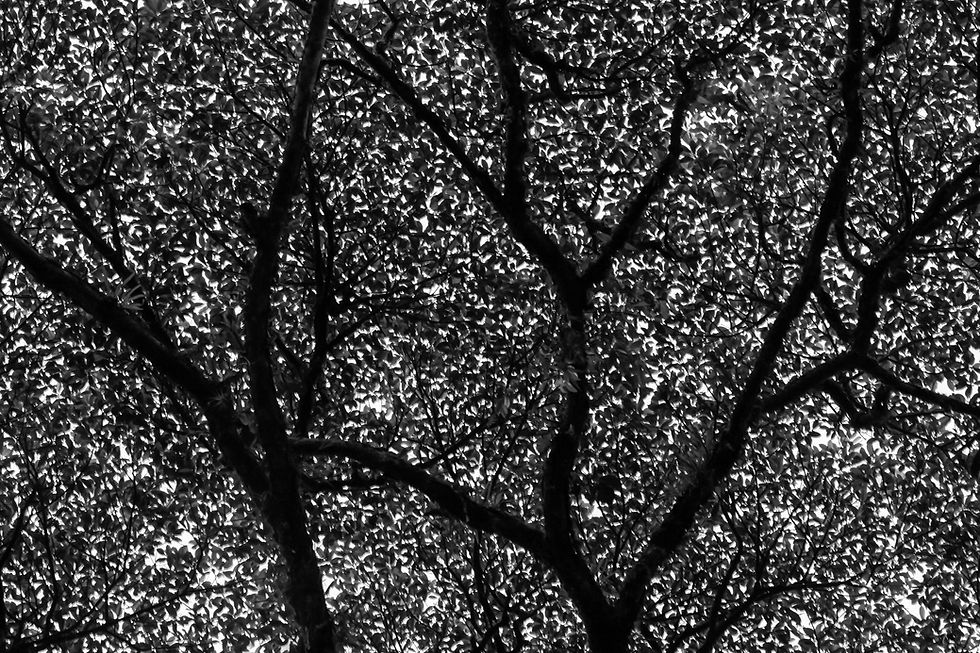
Decision trees are a powerful and widely used machine learning algorithm that falls under the category of supervised learning. They are commonly employed for classification and regression tasks and are known for their simplicity, interpretability, and versatility. Decision trees are used in various fields, including finance, healthcare, marketing, and more, making them one of the fundamental tools in a data scientist's toolkit.
In this post, we will delve into decision trees, how they work, and where they are commonly used. We'll also provide Python examples to illustrate their usage and discuss the advantages and disadvantages of decision trees compared to other machine learning methods.
How Decision Trees Work
Decision trees are a tree-like model that represents a decision-making process. At each node of the tree, a decision is made based on a feature, and the tree branches out into subsequent nodes. This process continues until a prediction or decision is made at a leaf node. Let's break down how decision trees work step by step:
Feature Selection
Decision trees start by selecting the most important feature from the dataset as the root node. This feature is chosen based on a criterion that measures how well it separates the data into different classes (for classification) or how well it predicts the target variable (for regression).
Splitting Criteria
At the heart of decision tree construction is the concept of splitting criteria. Decision trees partition the dataset into subsets based on the values of input features to make predictions. The most common splitting criteria for decision trees are:
Gini Impurity (for Classification): Gini impurity measures the level of disorder in a set of data points. For a set with multiple classes, the Gini impurity is calculated as follows:
Gini Impurity (I_Gini) = 1 - Σ (p_i)^2
where p_i represents the proportion of data points belonging to class i in the set.
The lower the Gini impurity, the purer the set, and it's desirable to minimize it during the tree construction.
Mean Squared Error (for Regression): Mean squared error (MSE) measures the variance of the target variable within a set of data points. For a set S, MSE is calculated as:
MSE(S) = Σ (y_i - ŷ)^2 / |S|
where y_i represents the actual target values, ŷ is the predicted value for the set, and |S| is the number of data points in set S. The objective in regression trees is to minimize MSE.
Building the Tree
Decision tree construction follows a recursive process:
Root Node: The initial feature is selected to split the data based on the chosen impurity measure. This feature is determined by evaluating the impurity reduction provided by each feature. The feature with the greatest reduction is selected as the root node.
Splitting Nodes: Subsets are created for each branch of the tree by partitioning the data based on the selected feature's values. This process is repeated for each node, leading to a tree structure.
Leaf Nodes: The process continues until one of the stopping criteria is met. A stopping criterion could be reaching a maximum depth, having a minimum number of data points in a node, or when impurity is below a certain threshold. At this point, the node becomes a leaf node, and a prediction is assigned.
Pruning
Pruning is a technique used to prevent overfitting in decision trees. Overfitting occurs when the tree is too deep and captures noise in the data. Pruning involves removing nodes from the tree that do not significantly contribute to its predictive power.
The mathematics behind pruning often involves the concept of cost-complexity pruning. The cost-complexity measure, denoted as α (alpha), quantifies the trade-off between the tree's complexity and its predictive power. The cost-complexity measure is defined as:
Cost-complexity (α) = Impurity(T) + α * Number of Terminal Nodes
Here, Impurity(T) represents the impurity of the tree T, and the second term represents a penalty for the number of terminal nodes. By adjusting the value of α, you can control the trade-off between tree complexity and predictive performance.
Entropy and Information Gain
Another important mathematical concept used in decision tree construction is entropy and information gain. Entropy measures the disorder or impurity in a set of data points. In the context of decision trees, entropy is used as an alternative to Gini impurity. For a set S with multiple classes, the entropy (H(S)) is calculated as:
H(S) = - Σ (p_i * log2(p_i))
where p_i represents the proportion of data points belonging to class i in set S. Information gain (IG) is a metric used to evaluate the reduction in entropy after a split. The higher the information gain, the better the split:
IG(S, F) = H(S) - Σ (|S_v| / |S|) * H(S_v)
where S is the parent set, F is the feature used for the split, and S_v represents the subsets created by the split.
Example in Python
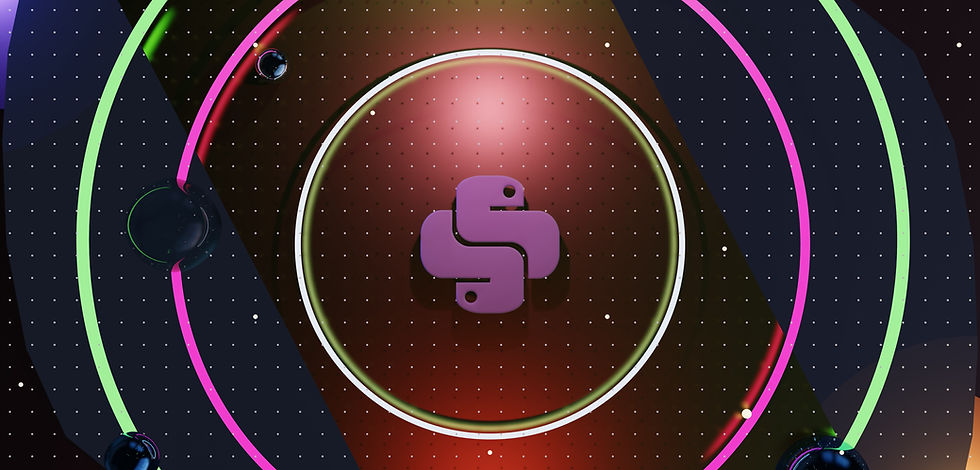
Let's consider a simple example using Python and the popular scikit-learn library to build a decision tree for a classification problem. We'll use the Iris dataset, which is a well-known dataset containing three classes of iris plants based on four features: sepal length, sepal width, petal length, and petal width.
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load the Iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a decision tree classifier
clf = DecisionTreeClassifier()
# Fit the model to the training data
clf.fit(X_train, y_train)
# Make predictions on the test data
y_pred = clf.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
In this example, we first load the Iris dataset, split it into training and testing sets, create a decision tree classifier, fit the model to the training data, and finally, evaluate its accuracy on the test data.
Where Decision Trees Are Used
Decision trees are applied in various domains and scenarios due to their versatility and simplicity. Here are some common areas where decision trees find application:
1. Finance: Credit Scoring
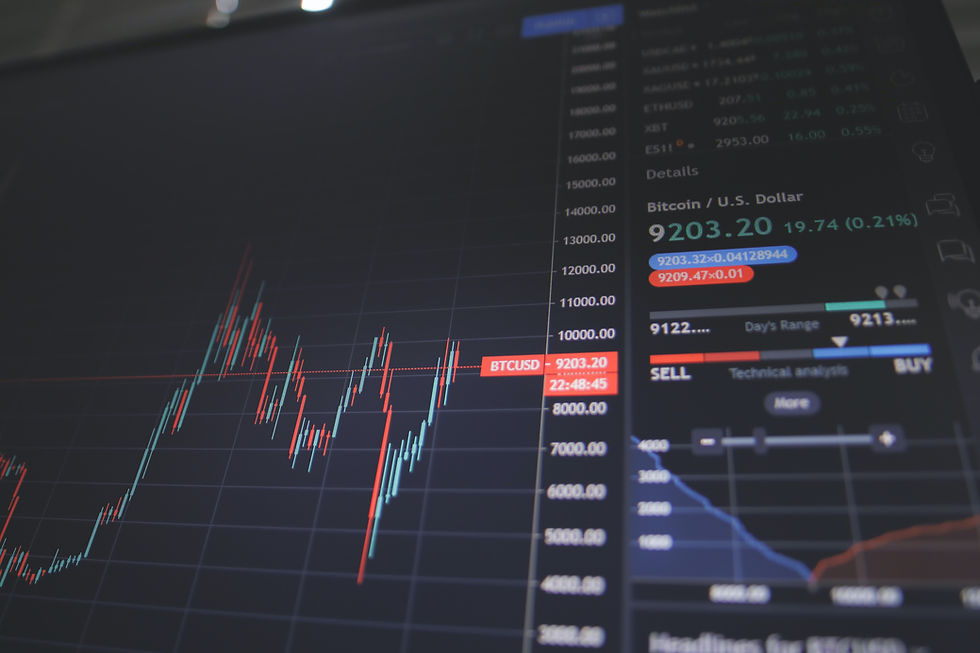
Use Case: Decision trees are commonly used in the financial industry for credit scoring. Lending institutions use these models to assess the creditworthiness of individuals or businesses applying for loans or credit.
Example: Suppose a bank wants to determine whether a new loan applicant is likely to default on their loan. They can use a decision tree model to evaluate the applicant's credit history, income, outstanding debts, and other financial attributes. The decision tree can be trained on historical data where the loan outcomes (default or non-default) are known. It then uses this information to make predictions for new applicants.
2. Healthcare: Medical Diagnosis

Use Case: In healthcare, decision trees are employed for medical diagnosis, assisting doctors in making decisions based on patient data, including symptoms, test results, and medical history.
Example: Let's consider a scenario where a decision tree is used to diagnose a patient's illness. The decision tree considers symptoms such as fever, cough, and chest pain, along with diagnostic test results like X-rays and blood tests. Based on this information, it can provide recommendations for the most likely diagnosis, such as "Common Cold," "Bronchitis," or "Pneumonia."
3. Marketing: Customer Segmentation

Use Case: Decision trees play a role in marketing by helping businesses segment their customer base. This enables targeted marketing strategies and better understanding of customer behavior.
Example: An e-commerce company wants to segment its customers for personalized marketing campaigns. They can create a decision tree model using features like purchase history, website interaction, and demographics. The decision tree may classify customers into segments like "Frequent Shoppers," "Discount Seekers," or "Window Shoppers," allowing the company to tailor marketing efforts to each group's preferences and behavior.
4. Manufacturing: Quality Control
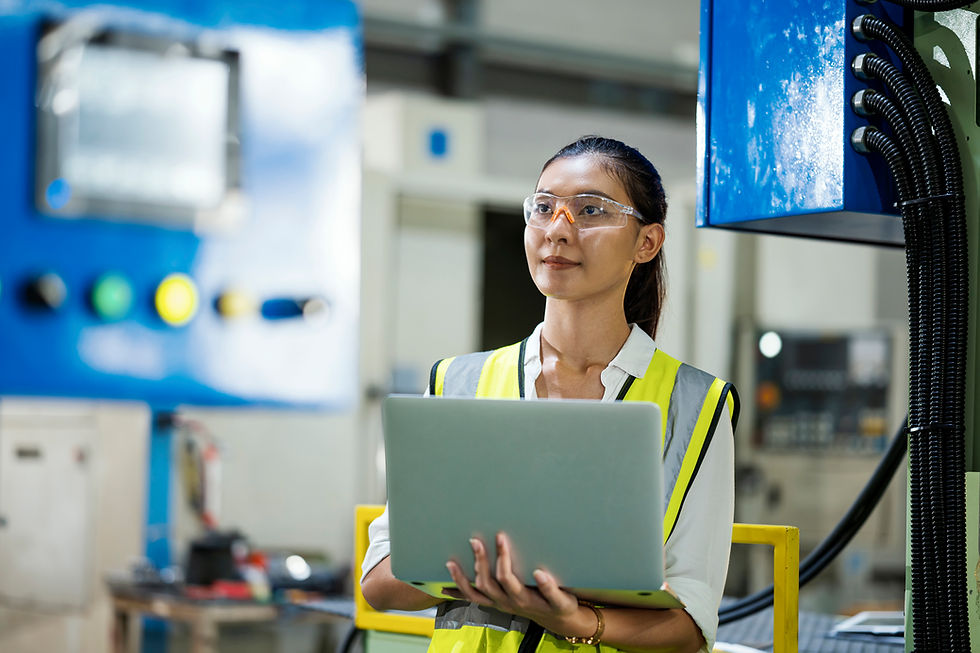
Use Case: In manufacturing, decision trees can be used for quality control to identify defects or issues in the production process.
Example: An automobile manufacturing plant is inspecting vehicles for defects before they are shipped to dealers. A decision tree model can be created to analyze various features of the vehicle, such as paint quality, alignment, and engine performance. The decision tree helps determine whether the vehicle should be classified as "Pass" or "Fail" based on these features.
5. Natural Language Processing (NLP): Sentiment Analysis
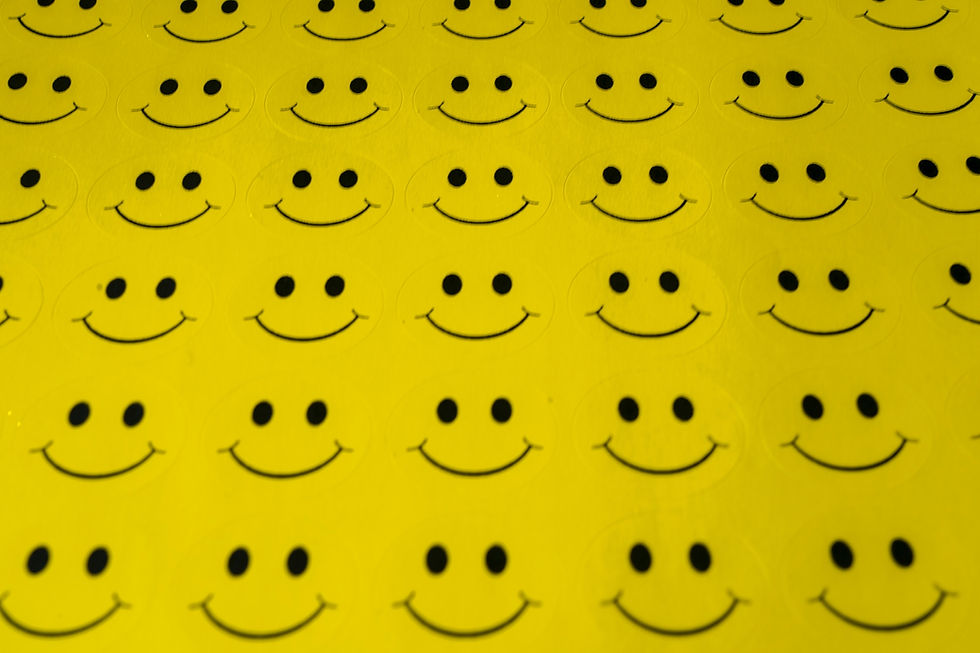
Use Case: In NLP, decision trees are utilized for sentiment analysis, which involves determining the sentiment (positive, negative, neutral) in text data, such as product reviews or social media comments.
Example: An e-commerce company wants to analyze customer reviews to gauge product satisfaction. They build a decision tree model that considers various textual features, such as keywords, sentiment words, and review length. The decision tree classifies the reviews into "Positive," "Negative," or "Neutral" sentiments, helping the company understand customer feedback.
6. Recommendation Systems: Collaborative Filtering
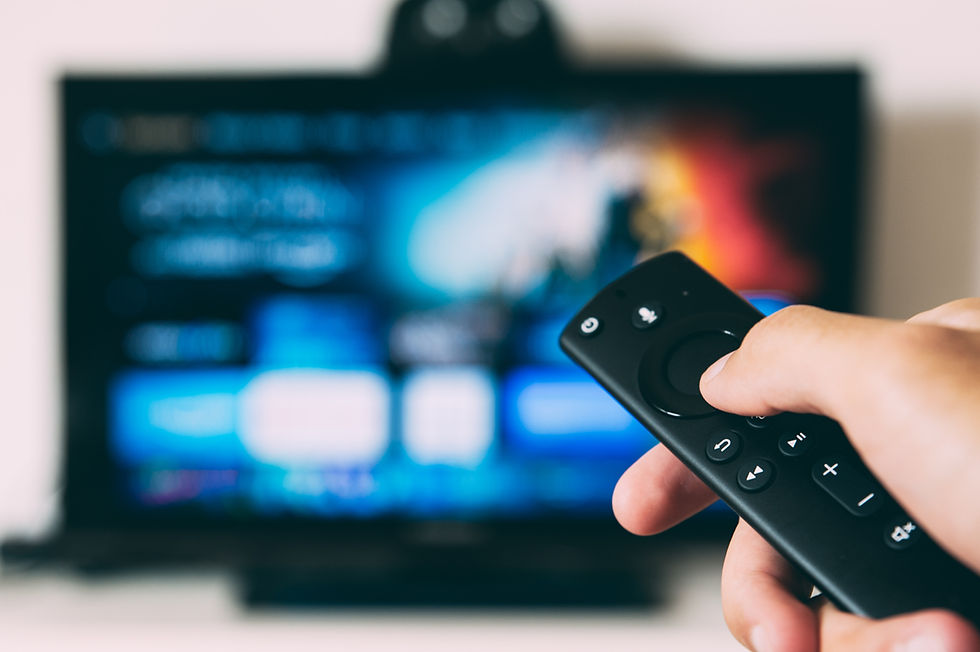
Use Case: Decision trees can be part of recommendation systems, particularly in collaborative filtering. Collaborative filtering is a technique that makes automatic predictions about a user's interests by collecting preferences from many users (collaborating).
Example: An online streaming platform wants to recommend movies to its users. The decision tree model uses historical user data to determine which movies are likely to be enjoyed by a particular user based on their past ratings and the ratings of other similar users. It can recommend movies by categorizing them into "Highly Recommended," "Recommended," or "Not Recommended."
These examples demonstrate the versatility of decision trees in various domains. They can be adapted to a wide range of applications by tailoring the choice of features and target variables. Moreover, decision trees provide an intuitive way to understand the decision-making process, making them a valuable tool for businesses and professionals seeking transparent and interpretable machine learning models.
Pros and Cons of Decision Trees
Pros:
Interpretability: Decision trees are easy to interpret and understand. They can be visualized, making it clear how a decision is made at each step.
Versatility: Decision trees can be used for both classification and regression tasks.
Handling Non-linearity: They can handle non-linear relationships between features and the target variable.
Feature Importance: Decision trees can provide information on the importance of each feature in the model's decision-making process.
No Assumptions About Data: Decision trees don't make assumptions about the distribution of data, making them robust to various types of data.
Handling Missing Data: They can handle missing values in the dataset, although methods for this may vary.
Cons:
Overfitting: Decision trees are prone to overfitting, especially when they become too deep and complex. This can lead to poor generalization on new data.
Instability: Small changes in the data can result in significantly different tree structures, making them unstable.
Bias Toward Dominant Classes: In classification tasks, if one class dominates the dataset, the decision tree may be biased toward that class.
Limited Expressiveness: Decision trees are not suitable for capturing complex patterns in data, as they are based on binary decisions at each node.
Comparison with Other Methods
Let's briefly compare decision trees with other commonly used machine learning methods:
Random Forests: Random forests are an ensemble method that builds multiple decision trees and combines their predictions. They are more robust and less prone to overfitting than individual decision trees.
Support Vector Machines (SVM): SVMs are powerful for classification tasks, especially when dealing with high-dimensional data. They can handle complex decision boundaries.
Neural Networks: Neural networks, especially deep learning models, can capture highly complex patterns and relationships in data. They are suitable for tasks where decision trees may fall short.
Naive Bayes: Naive Bayes is a simple yet effective method for text classification and spam detection. It's based on probabilistic principles.
k-Nearest Neighbors (KNN): KNN is used for classification and regression. It relies on the similarity between data points in the feature space.
In summary, decision trees are a valuable tool in the machine learning toolkit due to their interpretability and versatility. However, they come with limitations such as overfitting and a bias toward dominant classes. Depending on the nature of the data and the specific task, other algorithms like random forests, SVMs, neural networks, or Naive Bayes may offer better performance.
In practice, the choice of algorithm often depends on the problem at hand and the available data. Decision trees, when used judiciously and in combination with pruning or ensemble techniques, can be a powerful and interpretable solution for many machine learning tasks.
Comentarios