Reinforcement Learning with the Actor-Critic Method: A Comprehensive Guide
- vazquezgz
- Oct 15, 2023
- 5 min read
Updated: May 20, 2024
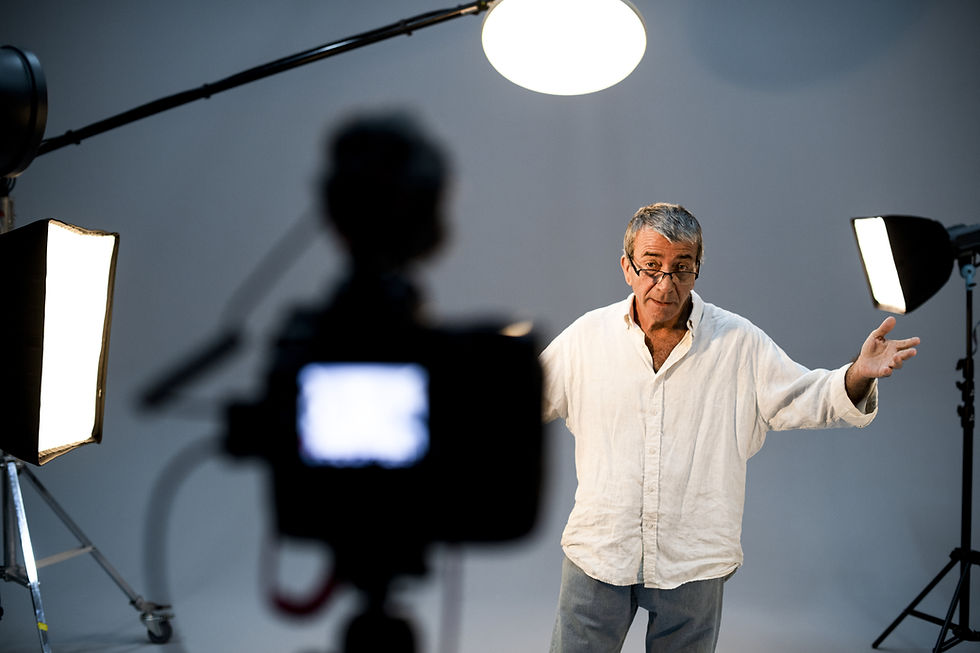
Reinforcement learning (RL) is a subfield of machine learning where an agent learns to make sequential decisions through interaction with an environment. One popular approach in reinforcement learning is the Actor-Critic method. This method combines the advantages of both value-based and policy-based RL techniques, making it a powerful tool for a wide range of applications. In this post, we will explore the Actor-Critic method, its applications, detailed workings, and provide Python examples to help you better understand this algorithm.
What is the Actor-Critic Method?
The Actor-Critic method is a reinforcement learning approach that combines the strengths of value-based methods and policy-based methods. It consists of two components: the Actor and the Critic.
Actor: The Actor is responsible for selecting actions. It defines a policy, often represented by a neural network, that maps states to actions. The policy is responsible for exploring and selecting actions that maximize expected rewards.
Critic: The Critic evaluates the actions taken by the Actor. It approximates the value function, which estimates the expected cumulative rewards when following a specific policy. The Critic helps the Actor learn which actions are better in a given state by providing feedback in the form of value functions.
How Actor-Critic Works:
Here's a step-by-step breakdown of how the Actor-Critic method operates:
Initialization: Initialize both the Actor and the Critic networks with random weights.
Data Collection: The Actor interacts with the environment, selecting actions according to its current policy. The Critic observes the state, action, and reward transitions.
Policy Improvement (Actor): The Actor updates its policy to maximize expected rewards. This is often done using policy gradients, where the policy is adjusted in the direction that increases the expected return. An example of this is the REINFORCE algorithm.
Value Estimation (Critic): The Critic uses the observed state, action, and reward transitions to estimate the value function. Common methods for this include the TD-learning and Q-learning approaches.
Policy Evaluation (Critic): The Critic evaluates the Actor's policy by providing feedback on how good the chosen actions are. This feedback guides the Actor in selecting better actions.
Parameter Updates: Both the Actor and Critic networks are updated iteratively. The Actor's policy is adjusted based on the Critic's evaluation, while the Critic's value estimates are updated based on the observed rewards and future value estimates.
Repeat: Steps 2-6 are repeated until the Actor's policy converges to an optimal policy or a satisfactory solution.
Applications of Actor-Critic:
The Actor-Critic method finds applications in various domains, including robotics, game playing, recommendation systems, and autonomous vehicles. Here are a few examples:
Game Playing: In the context of game playing, the Actor-Critic method has been successfully applied to train agents for playing video games, such as the popular game, "Dota 2."
Robotic Control: In robotics, the Actor-Critic approach is used for tasks like controlling robot arms, where the Actor controls the actions, and the Critic evaluates the results to fine-tune the policy.
Autonomous Driving: Autonomous vehicles often employ Actor-Critic techniques for decision-making. The Actor selects driving actions, and the Critic evaluates their safety and efficiency.
Finance: In finance, Actor-Critic can be applied to portfolio management, where the Actor decides on investments, and the Critic evaluates the performance of the portfolio over time.
Let's delve into four Python examples that illustrate how the Actor-Critic method can be implemented.
Example 1: Simple Actor-Critic in Python
# Example 1: Simple Actor-Critic in Python
# Import necessary libraries
import numpy as np
# Define a simple actor network
class Actor:
def __init__(self, input_dim, output_dim):
self.weights = np.random.rand(input_dim, output_dim)
def select_action(self, state):
return np.dot(state, self.weights)
# Define a simple critic network
class Critic:
def __init__(self, input_dim):
self.weights = np.random.rand(input_dim)
def evaluate(self, state):
return np.dot(state, self.weights)
# Instantiate actor and critic
actor = Actor(2, 1)
critic = Critic(2)
# Main training loop
for episode in range(1000):
state = np.random.rand(2)
action = actor.select_action(state)
reward = np.sum(state)
value = critic.evaluate(state)
# Update actor and critic
actor.weights += 0.01 * reward * action
critic.weights += 0.01 * (reward - value) * state
In this example, we create simple Actor and Critic classes to demonstrate the Actor-Critic concept.
Asynchronous Advantage Actor-Critic (A3C)
# Example 2: Asynchronous Advantage Actor-Critic (A3C)
import gym
import numpy as np
import tensorflow as tf
import multiprocessing
# Create the environment
env_name = 'CartPole-v1'
env = gym.make(env_name)
# Define the Actor and Critic networks using TensorFlow
class ActorCritic(tf.keras.Model):
def __init__(self, num_actions):
super(ActorCritic, self).__init__()
self.dense1 = tf.keras.layers.Dense(128, activation='relu')
self.policy_head = tf.keras.layers.Dense(num_actions, activation='softmax')
self.value_head = tf.keras.layers.Dense(1)
def call(self, inputs):
x = self.dense1(inputs)
return self.policy_head(x), self.value_head(x)
# Define the A3C worker
def worker(worker_id, global_actor_critic, optimizer, global_step):
local_actor_critic = ActorCritic(num_actions=env.action_space.n)
while global_step < max_global_steps:
state = env.reset()
done = False
episode_reward = 0
episode_length = 0
while not done:
with tf.GradientTape() as tape:
policy, value = local_actor_critic(state.reshape([1, -1]))
action = np.random.choice(env.action_space.n, p=np.squeeze(policy))
next_state, reward, done, _ = env.step(action)
episode_reward += reward
episode_length += 1
next_state = next_state.reshape([1, -1])
_, next_value = local_actor_critic(next_state)
if done:
target = reward
else:
target = reward + 0.99 * next_value
td_error = target - value
actor_loss = -tf.math.log(policy[0, action]) * td_error
critic_loss = td_error ** 2
actor_gradients = tape.gradient(actor_loss, local_actor_critic.trainable_variables)
critic_gradients = tape.gradient(critic_loss, local_actor_critic.trainable_variables)
optimizer.apply_gradients(zip(actor_gradients, global_actor_critic.trainable_variables))
optimizer.apply_gradients(zip(critic_gradients, global_actor_critic.trainable_variables))
global_step.assign_add(1)
if global_step % 100 == 0:
print(f'Worker {worker_id}, Step: {global_step}, Episode Reward: {episode_reward}')
state = next_state
# Define the hyperparameters
num_workers = 8
max_global_steps = 100000
# Create the global Actor-Critic network and optimizer
global_actor_critic = ActorCritic(num_actions=env.action_space.n)
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
global_step = tf.Variable(0)
# Create and start worker processes
processes = []
for i in range(num_workers):
p = multiprocessing.Process(target=worker, args=(i, global_actor_critic, optimizer, global_step))
processes.append(p)
p.start()
# Wait for all processes to finish
for p in processes:
p.join()
In this example, we implement Asynchronous Advantage Actor-Critic (A3C), a more advanced variant of the Actor-Critic method, to solve the CartPole problem using multiple worker processes.
Conclusion:
The Actor-Critic method is a versatile approach in reinforcement learning that combines the advantages of both policy-based and value-based methods. It offers several benefits, such as the ability to handle continuous action spaces, efficient exploration, and the potential for handling high-dimensional state spaces. However, it also has some drawbacks, including increased complexity in implementation and the need for careful hyperparameter tuning.
In comparison to other RL methods, Actor-Critic has the advantage of striking a balance between exploration and exploitation, making it suitable for complex tasks. It is often favored when working with continuous action spaces, and it can be extended to more advanced variants like A3C, which provides better sample efficiency.
Some of the limitations of Actor-Critic include sensitivity to hyperparameters, occasional instability in training, and the need for careful initialization. In cases where you have discrete action spaces and can afford high computation, Q-learning methods like DQN might be more straightforward to implement.
Ultimately, the choice of reinforcement learning method depends on the specific problem at hand and the available resources. Actor-Critic, with its balance between value estimation and policy optimization, remains a valuable tool in the RL toolkit for a wide range of applications.
Commenti