Reinforcement Learning with Deep Q-Networks (DQNs)
- vazquezgz
- Oct 8, 2023
- 4 min read
Updated: Mar 4, 2024
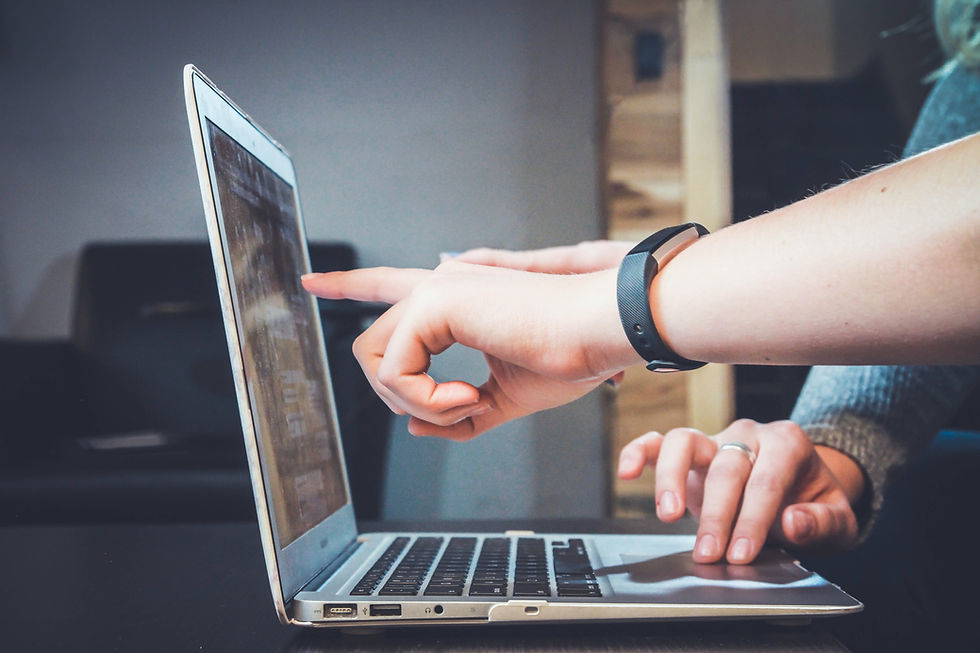
Reinforcement learning is a subfield of machine learning that focuses on training agents to make sequential decisions in an environment to maximize a cumulative reward. One of the most significant breakthroughs in reinforcement learning in recent years has been the development of Deep Q-Networks (DQNs). DQNs combine reinforcement learning with deep neural networks, making it possible to solve complex tasks in various domains. In this post, we will introduce DQNs, explain how they work in detail, provide four Python examples using publicly available datasets, and conclude with insights into their utility.
Introduction to Deep Q-Networks (DQNs)
Deep Q-Networks (DQNs) are a type of neural network architecture used in reinforcement learning. They were introduced by Volodymyr Mnih et al. in their seminal paper, "Playing Atari with Deep Reinforcement Learning" (2013), and have since become a cornerstone of deep reinforcement learning.
DQNs combine the power of deep neural networks with Q-learning, a traditional reinforcement learning technique. The fundamental idea behind DQNs is to approximate the Q-function, which represents the expected cumulative reward an agent can achieve from a given state and action. By using deep neural networks, DQNs can handle high-dimensional state spaces, making them suitable for tasks like playing video games, robotics, and autonomous driving.
How DQNs Work
Q-Learning Recap
Before diving into DQNs, let's briefly recap Q-learning, the underlying concept. Q-learning is a model-free reinforcement learning algorithm that learns a value function (Q-function) to estimate the expected cumulative reward for taking a specific action in a particular state. The Q-value is updated iteratively using the Bellman equation:
Q(s, a) = Q(s, a) + α * [r + γ * max(Q(s', a')) - Q(s, a)]
Where:
Q(s, a) is the Q-value for state s and action a.
α is the learning rate (step size).
r is the immediate reward after taking action a in state s.
γ is the discount factor, representing the importance of future rewards.
s' is the next state, and a' is the action taken in the next state.
DQNs: Approximating Q-Functions
1. State Representation and Preprocessing
The success of DQNs largely depends on the representation of the state. For tasks like playing video games, the input to the DQN is often raw pixel data from the game screen. However, raw pixel data can be extremely high-dimensional and noisy, making it challenging for traditional neural networks to handle efficiently.
To address this issue, preprocessing is typically applied to the raw state observations. Common preprocessing steps include:
Resizing: Scaling down the image to a smaller size, reducing computational complexity.
Grayscale Conversion: Converting color images to grayscale can reduce the input dimensionality.
Stacking Frames: Storing multiple consecutive frames as input helps capture motion information.
Normalization: Scaling pixel values to a smaller range, like [0, 1] or [-1, 1].
These preprocessing steps ensure that the DQN receives compact and informative state representations.
2. Q-Value Prediction with Neural Networks
The core component of a DQN is its neural network, which approximates the Q-function. This network takes the preprocessed state as input and outputs Q-values for each possible action. The architecture typically consists of convolutional layers to capture spatial patterns in the input, followed by fully connected layers for value estimation.
Here's a simplified Python code snippet demonstrating the Q-network architecture using the popular deep learning library TensorFlow/Keras:
import tensorflow as tf
from tensorflow.keras import layers, models
# Create a Q-network model
def create_q_network(input_shape, num_actions):
model = models.Sequential([
layers.Conv2D(32, (8, 8), strides=(4, 4), activation='relu', input_shape=input_shape),
layers.Conv2D(64, (4, 4), strides=(2, 2), activation='relu'),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(512, activation='relu'),
layers.Dense(num_actions) # Output layer with num_actions units
])
return model
3. Action Selection and Exploration
To choose actions, the DQN employs a strategy that balances exploration (trying new actions) and exploitation (choosing actions with the highest Q-values). A common approach is epsilon-greedy exploration, where the agent selects the best-known action with probability 1 - ε and explores with probability ε by selecting a random action.
import numpy as np
# Epsilon-greedy action selection
def epsilon_greedy(q_values, epsilon):
if np.random.rand() < epsilon:
# Explore: Choose a random action
return np.random.randint(len(q_values))
else:
# Exploit: Choose the action with the highest Q-value
return np.argmax(q_values)
4. Experience Replay
Experience replay is a crucial technique used in DQN training to improve sample efficiency and stabilize learning. Instead of using each experience immediately after it's collected, experiences (state, action, reward, next state) are stored in a replay buffer.
During training, batches of experiences are randomly sampled from this buffer, breaking the temporal correlations in the data. This approach prevents the DQN from overfitting to recent experiences and can lead to more stable and faster convergence.
from collections import deque
# Experience replay buffer
class ReplayBuffer:
def __init__(self, buffer_size):
self.buffer = deque(maxlen=buffer_size)
def add(self, experience):
self.buffer.append(experience)
def sample(self, batch_size):
batch = random.sample(self.buffer, batch_size)
return batch
5. Q-Value Update and Target Network
The Q-value update step is based on the Bellman equation:
Q(s, a) = Q(s, a) + α * [r + γ * max(Q(s', a')) - Q(s, a)]
In practice, the DQN uses a target network to stabilize training. This target network is a copy of the Q-network and is updated less frequently. The Q-value update uses this target network to calculate the target Q-values:
# Q-value update using target network
def update_q_network(q_network, target_network, experiences, gamma, alpha):
# Extract experiences
states, actions, rewards, next_states, dones = zip(*experiences)
# Calculate target Q-values using the target network
target_q_values = q_network.predict(np.array(next_states))
max_target_q_values = np.max(target_q_values, axis=1)
targets = []
for i in range(len(experiences)):
if dones[i]:
targets.append(rewards[i])
else:
targets.append(rewards[i] + gamma * max_target_q_values[i])
targets = np.array(targets)
# Update the Q-network using the calculated targets
q_network.fit(np.array(states), targets, epochs=1, verbose=0)
This approach ensures that the target Q-values are more stable during training, leading to improved convergence.
Deep Q-Networks (DQNs) represent a significant advancement in reinforcement learning, allowing agents to tackle complex tasks by approximating the Q-function using deep neural networks. They have been applied successfully in various domains, from playing video games to controlling robots and autonomous vehicles. Understanding the key components of DQNs, such as state preprocessing, neural network architecture, exploration strategies, experience replay, and target networks, is crucial for effectively implementing and training these models. As reinforcement learning continues to evolve, DQNs are expected to remain a pivotal tool for solving increasingly challenging problems in artificial intelligence.
Comments