Exploring Unsupervised Learning Methods: An In-Depth Guide
- vazquezgz
- Oct 2, 2023
- 3 min read
Updated: Mar 4, 2024
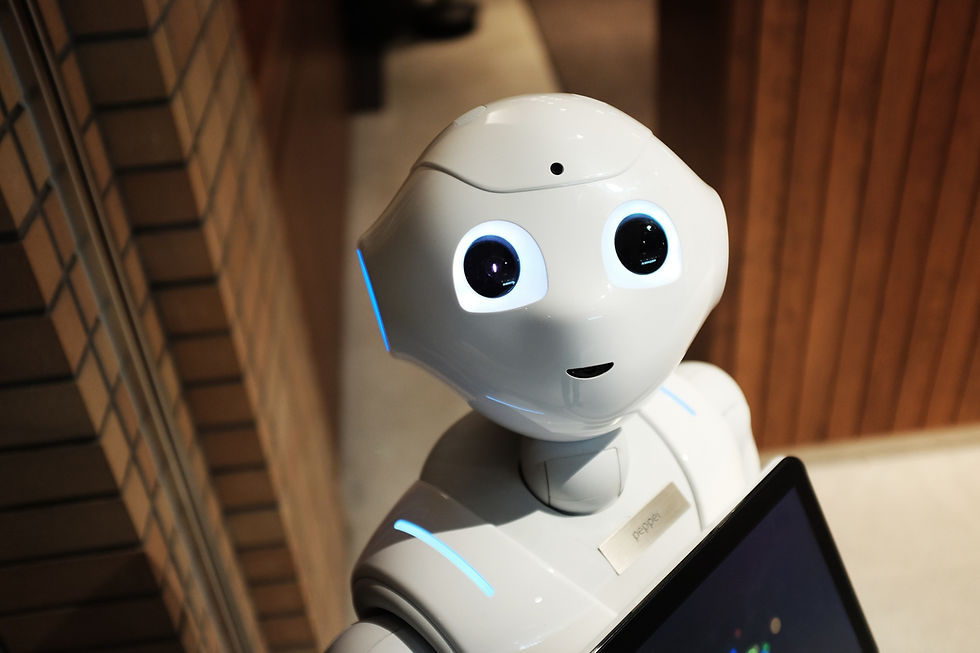
Unsupervised learning is a fascinating field of machine learning where algorithms aim to discover patterns and structures within datasets without the presence of explicit labels or target variables. Instead of being provided with labeled data, unsupervised learning algorithms rely on inherent data characteristics to extract meaningful insights. In this comprehensive guide, we will delve into various common unsupervised learning methods, explore how they work, provide Python examples, compare their performance using the Iris flower dataset, and discuss their advantages and disadvantages.
Overview of Common Unsupervised Learning Methods:
1. K-Means Clustering:
How it Works: K-Means is a partitioning algorithm that divides a dataset into 'k' clusters, aiming to minimize the sum of squared distances between data points and the centroids of their respective clusters.
Common Usage: K-Means is widely used in customer segmentation, image compression, and anomaly detection.
Python Example:
from sklearn.cluster import KMeans
# Load the Iris dataset
from sklearn.datasets import load_iris
data = load_iris()
X = data.data
# Fit K-Means to the data
kmeans = KMeans(n_clusters=3)
kmeans.fit(X)
2. Hierarchical Clustering:
How it Works: Hierarchical clustering builds a tree-like structure of data points, forming clusters at different levels of granularity. It is represented as a dendrogram.
Common Usage: Hierarchical clustering is used in biology for taxonomy, image analysis, and document clustering.
Python Example:
from scipy.cluster.hierarchy import dendrogram, linkage
import matplotlib.pyplot as plt
# Calculate linkage matrix
Z = linkage(X, 'ward')
# Plot the dendrogram
dendrogram(Z)
plt.show()
3. Principal Component Analysis (PCA):
How it Works: PCA is a dimensionality reduction technique that transforms the data into a new coordinate system where each dimension (principal component) captures the most variance.
Common Usage: PCA is applied in feature reduction, image compression, and noise reduction.
Python Example:
from sklearn.decomposition import PCA
# Fit PCA to the data
pca = PCA(n_components=2)
X_pca = pca.fit_transform(X)
4. Gaussian Mixture Models (GMM):
How it Works: GMM assumes that the data is generated by a mixture of several Gaussian distributions. It estimates the parameters of these distributions to fit the data.
Common Usage: GMM is used in image segmentation, fraud detection, and speech recognition.
Python Example:
from sklearn.mixture import GaussianMixture
# Fit GMM to the data
gmm = GaussianMixture(n_components=3)
gmm.fit(X)
5. t-Distributed Stochastic Neighbor Embedding (t-SNE):
How it Works: t-SNE is a dimensionality reduction technique that focuses on preserving pairwise similarities between data points in a lower-dimensional space.
Common Usage: t-SNE is valuable for visualization and exploratory data analysis.
Python Example:
from sklearn.manifold import TSNE
# Fit t-SNE to the data
tsne = TSNE(n_components=2)
X_tsne = tsne.fit_transform(X)
Method Comparisons on the Iris Dataset:
To compare the methods, we will use, once again, the Iris flower dataset. We'll evaluate their performance in terms of cluster separation and visualization using techniques like Silhouette Score and scatter plots. Please note that in practice, we would use unsupervised methods when the true labels are not available.
Advantages and Disadvantages:
K-Means Clustering:
Advantages:
Simple and computationally efficient.
Works well on spherical clusters.
Disadvantages:
Requires specifying the number of clusters (k).
Sensitive to initial centroid placement.
Hierarchical Clustering:
Advantages:
Produces a hierarchy of clusters at different levels.
No need to specify the number of clusters in advance.
Disadvantages:
Computationally intensive for large datasets.
Principal Component Analysis (PCA):
Advantages:
Reduces dimensionality while preserving most of the variance.
Useful for feature selection and visualization.
Disadvantages:
Assumes linear relationships between variables.
Gaussian Mixture Models (GMM):
Advantages:
More flexible than K-Means, can handle different cluster shapes.
Provides probabilistic cluster assignments.
Disadvantages:
Sensitive to initialization.
Can converge to local optima.
t-Distributed Stochastic Neighbor Embedding (t-SNE):
Advantages:
Excellent for visualizing high-dimensional data.
Preserves pairwise similarities.
Disadvantages:
Random initialization can lead to different embeddings.
Unsupervised learning methods offer powerful tools to uncover hidden patterns and structures in data. Each method has its own strengths and weaknesses, making them suitable for various applications. In this guide, we explored K-Means clustering, hierarchical clustering, PCA, Gaussian Mixture Models, and t-SNE, and compared their performance on the Iris dataset. In our next post, we will delve into the exciting world of reinforcement learning, where agents learn to make decisions through interaction with their environment. Stay tuned for more insights into the realm of machine learning!
Comments