A Beginner's Guide to Data Preprocessing in Machine Learning and AI
- vazquezgz
- Sep 25, 2023
- 5 min read
Updated: Mar 4, 2024
Welcome back to our journey through the fascinating world of Machine Learning and Artificial Intelligence. In this chapter, we'll dive into the crucial step of data preprocessing. We'll explore how to handle missing data, deal with categorical variables, partition datasets, and select meaningful features. To make it easier for beginners, we'll use Python libraries like Scikit-Learn and Pandas and provide plenty of examples.
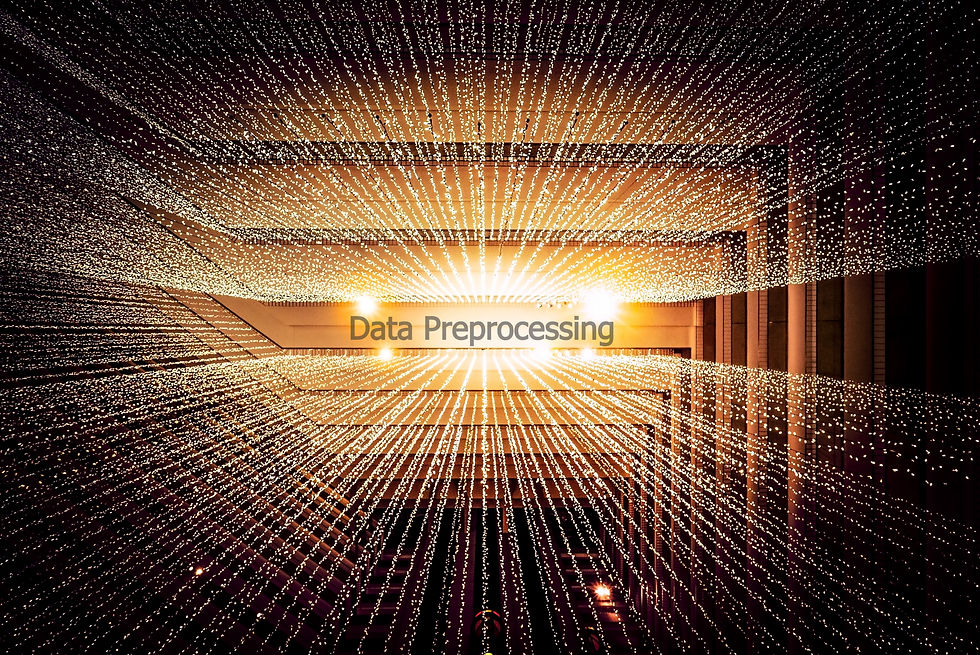
Handling Missing Data:
Dealing with missing data is a common challenge in machine learning. Fortunately, Scikit-Learn and Pandas provide tools to handle this issue, SimpleImputer.
import pandas as pd
from sklearn.impute import SimpleImputer
# Load your dataset
data = pd.read_csv('your_dataset.csv')
# Initialize the imputer
imputer = SimpleImputer(strategy='mean')
# Fill missing values with the mean of the column
data['column_with_missing_data'] = imputer.fit_transform(data[['column_with_missing_data']])
Handling Categorical Data:
Handling categorical data is a crucial aspect of data preprocessing in machine learning. Categorical data represents discrete values that belong to specific categories or groups. Unlike numerical data, which can be directly used in many machine learning algorithms, categorical data needs to be transformed into a numerical format for most algorithms to work effectively. This process is known as "categorical data encoding" or "categorical feature encoding."
Here are some common methods for handling categorical data in machine learning:
Label Encoding: Label encoding involves assigning a unique numerical label to each category in a categorical feature. This method is suitable for ordinal data where the order of categories matters. However, it may not be suitable for nominal data (categories with no inherent order).
from sklearn.preprocessing import LabelEncoder
data = ["cat", "dog", "fish", "dog", "cat"]
encoder = LabelEncoder()
encoded_data = encoder.fit_transform(data)
print(encoded_data)
Output:
[0 1 2 1 0]
One-Hot Encoding: One-hot encoding creates binary columns for each category in a categorical feature. Each column represents a category, and only one column has a value of 1 while the others are 0 for each data point. This method is suitable for nominal data.
import pandas as pd
data = ["red", "blue", "green", "red", "blue"]
one_hot_encoded = pd.get_dummies(data)
print(one_hot_encoded)
Output:
blue green red
0 0 0 1
1 1 0 0
2 0 1 0
3 0 0 1
4 1 0 0
Binary Encoding: Binary encoding is a compromise between label encoding and one-hot encoding. It first assigns numerical values to categories, then converts those values to binary code. This method can be useful for high-cardinality categorical features.
import category_encoders as ce
import pandas as pd
data = ["cat", "dog", "fish", "dog", "cat"]
encoder = ce.BinaryEncoder(cols=['animal'])
encoded_data = encoder.fit_transform(pd.DataFrame({'animal': data}))
print(encoded_data)
Output:
animal_0 animal_1 animal_2
0 0 0 1
1 0 1 0
2 0 1 1
3 0 1 0
4 0 0 1
Target Encoding: Target encoding uses the target variable's mean or other statistics to encode categorical features. This can be useful when there is a relationship between the categorical feature and the target variable.
import pandas as pd
data = pd.DataFrame({'Category': ['A', 'B', 'A', 'C', 'B'], 'Target':[1, 2, 1, 3, 2]})
target_means = data.groupby('Category')['Target'].mean().to_dict()
data['Category_Encoded'] = data['Category'].map(target_means)
print(data)
Output:
Category Target Category_Encoded
0 A 1 1.0
1 B 2 2.0
2 A 1 1.0
3 C 3 3.0
4 B 2 2.0
Handling categorical data correctly is crucial for building accurate machine learning models, as improper encoding can lead to biased or incorrect predictions. The choice of encoding method depends on the nature of the data and the machine learning algorithm being used.
Partitioning the Dataset:
Partitioning the dataset is a fundamental step in machine learning project workflows. It involves splitting the available data into different subsets for specific purposes, primarily for training and evaluating machine learning models. Let's delve into the importance of dataset partitioning and discuss the details of dataset splitting using scikit-learn's train_test_split function.
Importance of Dataset Partitioning:
Model Training and Testing: The primary reason for partitioning a dataset is to separate it into two or more subsets: one for training and one or more for testing. This allows you to train your machine learning model on one portion of the data and evaluate its performance on another. This separation helps you estimate how well your model will perform on unseen data.
Avoiding Overfitting: By having a dedicated test set, you can assess whether your model generalizes well to new, unseen examples or if it has overfit the training data. Overfitting occurs when a model learns to memorize the training data instead of learning the underlying patterns.
Hyperparameter Tuning: During model development, you may need to fine-tune hyperparameters. A validation set is often used for this purpose. It allows you to experiment with different hyperparameters without contaminating the test set's results.
Cross-Validation: In addition to a single train-test split, partitioning into multiple folds for cross-validation helps you assess model stability and performance across different data subsets. It's particularly useful when the dataset is limited.
Using train_test_split from scikit-learn:
Scikit-learn provides a convenient function called train_test_split for partitioning datasets into training and testing sets. Here's how to use it:
from sklearn.model_selection import train_test_split
# Splitting data into features (X) and target (y)
X = dataset.drop(columns=['target_column'])
y = dataset['target_column']
# Splitting into training (80%) and testing (20%)
sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Optional: Splitting a validation set from the training data X_train, X_val, y_train, y_val = train_test_split(X_train, y_train, test_size=0.2, random_state=42)
In the code above:
X represents the feature matrix, while y represents the target variable.
test_size determines the proportion of data to allocate for testing. In this example, 20% of the data is reserved for testing.
random_state is used to ensure reproducibility; setting it to a specific value ensures that the same split is generated every time you run the code.
Cross-Validation with KFold from scikit-learn:
For more advanced partitioning and cross-validation, you can use scikit-learn's KFold or StratifiedKFold classes. These allow you to perform k-fold cross-validation, which is useful for assessing model performance across multiple splits of the data.
from sklearn.model_selection import KFold
# Example of 5-fold cross-validation
kf = KFold(n_splits=5, shuffle=True, random_state=42)
for train_index, test_index in kf.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
In the code above, KFold creates five different train-test splits, each containing 80% of the data for training and 20% for testing. The shuffle parameter ensures that the data is randomly shuffled before splitting.
In summary, proper dataset partitioning is essential for building and evaluating machine learning models effectively. Scikit-learn provides versatile tools like train_test_split and KFold to assist with this process, allowing you to assess model performance, avoid overfitting, and fine-tune hyperparameters with confidence.
Selecting Meaningful Features:
Feature selection helps us choose the most important features for our model, reducing complexity and improving performance. Let's explore L1 and L2 regularization as methods to achieve this.
from sklearn.linear_model import Ridge
# Create a Ridge Regression model with L2 regularization
model = Ridge(alpha=1.0)
# Fit the model to your training data
model.fit(X_train, y_train)
Geometric Interpretation of L2 Regularization:
L2 regularization adds a penalty term to the loss function, encouraging smaller coefficient values. Geometrically, this shrinks the coefficient vectors towards the origin, preventing overfitting.
from sklearn.feature_selection import SequentialFeatureSelector
from sklearn.ensemble import RandomForestRegressor
# Create a RandomForestRegressor
model = RandomForestRegressor()
# Initialize SequentialFeatureSelector
selector = SequentialFeatureSelector(model, n_features_to_select=5, direction='backward')
# Fit the selector to your training data
selector.fit(X_train, y_train)
# Get the selected features
selected_features = X_train.columns[selector.get_support()]
Data preprocessing is a critical step in building successful machine learning models. We've covered how to handle missing data, deal with categorical variables, partition datasets, and select meaningful features using Python libraries like Scikit-Learn and Pandas. With these foundational skills, you're well on your way to mastering the art of machine learning and AI. Stay tuned for more exciting chapters on this journey!
Comments